Course: Kotlin Fundamentals for Mobile Development
duration: 23 hours |
Language: English (US) |
access duration: 180 days |

Details
In this course, you will learn about the Kotlin programming language and basic principles of programming with Kotlin. You will also receive additional tools on how to use Kotlin with Android app development.
You will gain insight into creating visually appealing layouts and navigation in Android applications, and you will learn how to build interactive layouts with GridView and ListView.
At the end of this course you will take an exam to test your knowledge of the Kotlin fundamentals.
Result
After this course, you will have gained a deeper understanding of the key principles within the Kotlin programming language. Additionally, you will have an overview on how to develop Android apps using Kotlin.
You will also learn more about app layouts, widgets, and navigation structures.
Prerequisites
No formal requirements. However, some prior knowledge of Java or other programming languages is recommended.
Target audience
Software Developer, Web Developer
Content
Kotlin Fundamentals for Mobile Development
Getting Started with Kotlin
Since 2019 Kotlin has been designated as the official programming language for Android development. However, Kotlin can be used for more than just mobile application development. Kotlin supports server-side development, full-stack development, data science, native application development, and multiplatform development. Kotlin compiles to Java bytecode, runs on the Java Virtual Machine, and can interoperate with existing Java code in your projects. In this course, you will be introduced to the Kotlin programming language. You will discover various use cases for Kotlin and identify when you would choose to use Kotlin over Java. You will use the Kotlin online playground to write your first bit of code, run Kotlin on the Java Virtual Machine (JVM), and compile Kotlin code to JavaScript. Next, you will use the Kotlin command line compiler on your local machine and run the Kotlin REPL (read-evaluate-print-loop) interactive environment to work with Kotlin code. Finally, you will install the IntelliJ integrated development environment to work with Kotlin and create and run your first Kotlin project.
Basic Programming Concepts in Kotlin
Kotlin supports the same basic concepts as other high-level programming languages such as Java and C#. However, Kotlin has a unique, more concise syntax than these languages and also has some additional features which make programming in Kotlin more robust and secure. In this course, you will learn to work with basic Kotlin data types and syntax. You will explore how to make a choice between val-type variables and var-type variables and the details of how to use those in your code. You will also use string templates to evaluate expressions embedded in strings. Next, you will work with conditional branching using if-else statements and expressions. You will perform multiple conditional checks using when statements and expressions, work with collection data structures such as lists, sets, and maps, and use looping constructs such as for-loops, while loops, and do-while loops. Finally, you will explore null safety features in Kotlin such as null-safe invocations, not-null assertions, and the Elvis operator.
Functional Programming with Kotlin
Kotlin supports functional and object-oriented programming constructs. Functions in Kotlin are first-class citizens and function objects can be treated just like other data types. In addition, Kotlin supports very concise function expressions called lambdas that are very often used with collection-related operations. In this course, you will learn the structure, syntax, and features of basic functions in Kotlin. You will learn to define default values for function input parameters, use named and positioned parameters with functions, and create functions with variable numbers of arguments. Next, you will work with higher-order functions. You will learn to pass in functions as input arguments to other functions and return functions as return values from functions. You will also implement and use closures in your code. Finally, you will define and use lambda expressions which are short, concise function definitions for use and throw functions. You will learn how to use the trailing lambda syntax in Kotlin and practice creating and using lambda expressions with collections.
Object-oriented Programming with Kotlin
A core feature of Kotlin is its ability to interoperate with Java code. Classes and objects in Kotlin are built on the same principles as in Java but Kotlin has additional features that improve upon Java's object-oriented model, including the ability to implement enum classes, sealed classes, data classes, and extension functions for third-party classes. In this course, you will be introduced to classes and objects in Kotlin. You will learn to define and use primary constructors, secondary constructors, and initializer blocks. You will also see how you can define custom getters and setters for class properties. Next, you will learn how you can inherit from classes in Kotlin and implement interfaces. You will study the implementation and use of functional interfaces and other special classes that Kotlin supports, such as enum classes, sealed classes, data classes, and companion objects. Finally, you will see how you can use extension functions to extend third-party class functionality and use coroutines for asynchronous programming.
Kotlin: Getting Started with Android App Development
Android application development remains highly relevant due to the widespread use of Android devices and the increasing demand for mobile applications. With the growing reliance on smartphones and tablets for various tasks, from communication and entertainment to productivity and e-commerce, Android apps play a vital role in meeting the evolving needs and preferences of users. In this course, you will embark on a journey to explore the essential concepts of Android development. You will learn about the four crucial components of Android applications - activities, services, broadcast receivers, and content providers. You will grasp the significance of the Android manifest file and gain familiarity with the fundamental architectural principles that will govern the development of robust Android applications. Next, you will learn how to set up and install Android Studio. You will create and configure an Android Views project and you will recognize the importance of selecting the appropriate Android API level to ensure compatibility and maximize the potential of your application. By exploring the various directories and folders within an Android project, you will gain a comprehensive understanding of their roles and functions. Finally, you will dive into the practical aspects of Android development by creating an Android Virtual Device (AVD) within Android Studio. This AVD will enable you to execute and evaluate your applications directly within the development environment. You will run your first application, fine-tune the layout, and manipulate the included resources, providing you with hands-on experience in developing apps using Android Studio.
Kotlin: Understanding Views & Layouts
Views and layouts are essential in Android applications as they determine the visual presentation, user interaction, and overall user experience, enabling developers to create intuitive and visually appealing interfaces that adapt to different device sizes and orientations. In this course you will learn the basics of using layouts or ViewGroups to design your application's screen. You will create screens using TextViews, EditText widgets, and ImageViews. Next, you will improve the look and feel of your application by including a MaterialToolbar to display the app title and other actions. You will also see how you can incorporate and wire up a floating action button in your app. Furthermore, you will explore listeners in more detail by developing a simple counter application that also includes a Snackbar for notifications. Finally, you will expand your skills in Android app development by exploring different layout options, including the linear, relative, frame, and constraint layouts.
Kotlin: Building Interactive Layouts Using GridView & ListView
Interactive dynamic layouts such as GridView and ListView in Android enable developers to present data in a visually appealing and interactive manner. These views leverage adapters as a bridge between the data source and the layout, allowing for improved performance using view recycling. In this course, we will create dynamic views using GridView and ListView layouts. We learn how to recycle views within the layouts, optimizing performance and memory usage by reusing existing views. Furthermore, we will explore formatting the layouts to utilize cards, enhancing the visual presentation of the content. Next, we will explore a variety of commonly used widgets in mobile applications. We will create an autocomplete text view, providing users with suggestions and auto-completion functionality for efficient input. We'll also create multi-autocomplete text views, empowering users to select multiple suggestions. We will also incorporate a ScrollView to allow users to navigate and view content that extends beyond the screen size. Finally, we will incorporate a toggle switch into our app, allowing users to easily switch between two states or options. We will create a ListView with checkboxes, enabling users to select multiple items from a list. Additionally, we will utilize the toggle button for state selection and create a screen with a popup menu, providing users with a visually organized menu of options or actions.
Kotlin: Working with Intents, Activities, & Fragments
Intents, activities, and fragments are essential components in Android development as they enable seamless navigation between screens, facilitate modular and reusable code structures, and allow for efficient communication and data transfer within an Android application. In this course, we will begin by exploring the functionality of intents in Android applications. Through hands-on exercises, we will learn to navigate between activities using intents, incorporating both implicit and explicit intents. We will also leverage intents to direct users to external web pages, enhancing the flow and navigation within our applications. Next, we will delve into advanced techniques in Android app development. We will set up the user interface for a reservations app and utilize intents to navigate to the reservations page, implementing view manipulation code to accept and display reservations. We will create view binding objects to streamline the process of accessing views. Additionally, we will use data binding to specify data directly within XML layouts for seamless integration. Then we will enhance list handling capabilities by leveraging RecyclerView. Finally, we will modularize our app by dividing it into fragments and pass input arguments to fragments for customization. We will learn to dynamically add and replace fragments using the fragment manager and fragment transactions and explore backstack management, enabling smooth back navigation within our app. To facilitate effective communication between activities and fragments, we will utilize ViewModel architecture, enhancing data exchange and interaction.
Final Exam: Kotlin Fundamentals
Final Exam: Kotlin Fundamentals will test your knowledge and application of the topics presented throughout the Kotlin Fundamentals track.
Course options
We offer several optional training products to enhance your learning experience. If you are planning to use our training course in preperation for an official exam then whe highly recommend using these optional training products to ensure an optimal learning experience. Sometimes there is only a practice exam or/and practice lab available.
Optional practice exam (trial exam)
To supplement this training course you may add a special practice exam. This practice exam comprises a number of trial exams which are very similar to the real exam, both in terms of form and content. This is the ultimate way to test whether you are ready for the exam.
Optional practice lab
To supplement this training course you may add a special practice lab. You perform the tasks on real hardware and/or software applicable to your Lab. The labs are fully hosted in our cloud. The only thing you need to use our practice labs is a web browser. In the LiveLab environment you will find exercises which you can start immediately. The lab enviromentconsist of complete networks containing for example, clients, servers,etc. This is the ultimate way to gain extensive hands-on experience.
Sign In
WHY_ICTTRAININGEN
Via ons opleidingsconcept bespaar je tot 80% op trainingen
Start met leren wanneer je wilt. Je bepaalt zelf het gewenste tempo
Spar met medecursisten en profileer je als autoriteit in je vakgebied.
Ontvang na succesvolle afronding van je cursus het officiële certificaat van deelname van Icttrainingen.nl
Krijg inzicht in uitgebreide voortgangsinformatie van jezelf of je medewerkers
Kennis opdoen met interactieve e-learning en uitgebreide praktijkopdrachten door gecertificeerde docenten
Orderproces
Once we have processed your order and payment, we will give you access to your courses. If you still have any questions about our ordering process, please refer to the button below.
read more about the order process
Een zakelijk account aanmaken
Wanneer u besteld namens uw bedrijf doet u er goed aan om aan zakelijk account bij ons aan te maken. Tijdens het registratieproces kunt u hiervoor kiezen. U heeft vervolgens de mogelijkheden om de bedrijfsgegevens in te voeren, een referentie en een afwijkend factuuradres toe te voegen.
Betaalmogelijkheden
U heeft bij ons diverse betaalmogelijkheden. Bij alle betaalopties ontvangt u sowieso een factuur na de bestelling. Gaat uw werkgever betalen, dan kiest u voor betaling per factuur.

Cursisten aanmaken
Als u een zakelijk account heeft aangemaakt dan heeft u de optie om cursisten/medewerkers aan te maken onder uw account. Als u dus meerdere trainingen koopt, kunt u cursisten aanmaken en deze vervolgens uitdelen aan uw collega’s. De cursisten krijgen een e-mail met inloggegevens wanneer zij worden aangemaakt en wanneer zij een training hebben gekregen.
Voortgangsinformatie
Met een zakelijk account bent u automatisch beheerder van uw organisatie en kunt u naast cursisten ook managers aanmaken. Beheerders en managers kunnen tevens voortgang inzien van alle cursisten binnen uw organisatie.
What is included?
Certificate of participation | Yes |
Monitor Progress | Yes |
Award Winning E-learning | Yes |
Mobile ready | Yes |
Sharing knowledge | Unlimited access to our IT professionals community |
Study advice | Our consultants are here for you to advice about your study career and options |
Study materials | Certified teachers with in depth knowledge about the subject. |
Service | World's best service |
Platform
Na bestelling van je training krijg je toegang tot ons innovatieve leerplatform. Hier vind je al je gekochte (of gevolgde) trainingen, kan je eventueel cursisten aanmaken en krijg je toegang tot uitgebreide voortgangsinformatie.
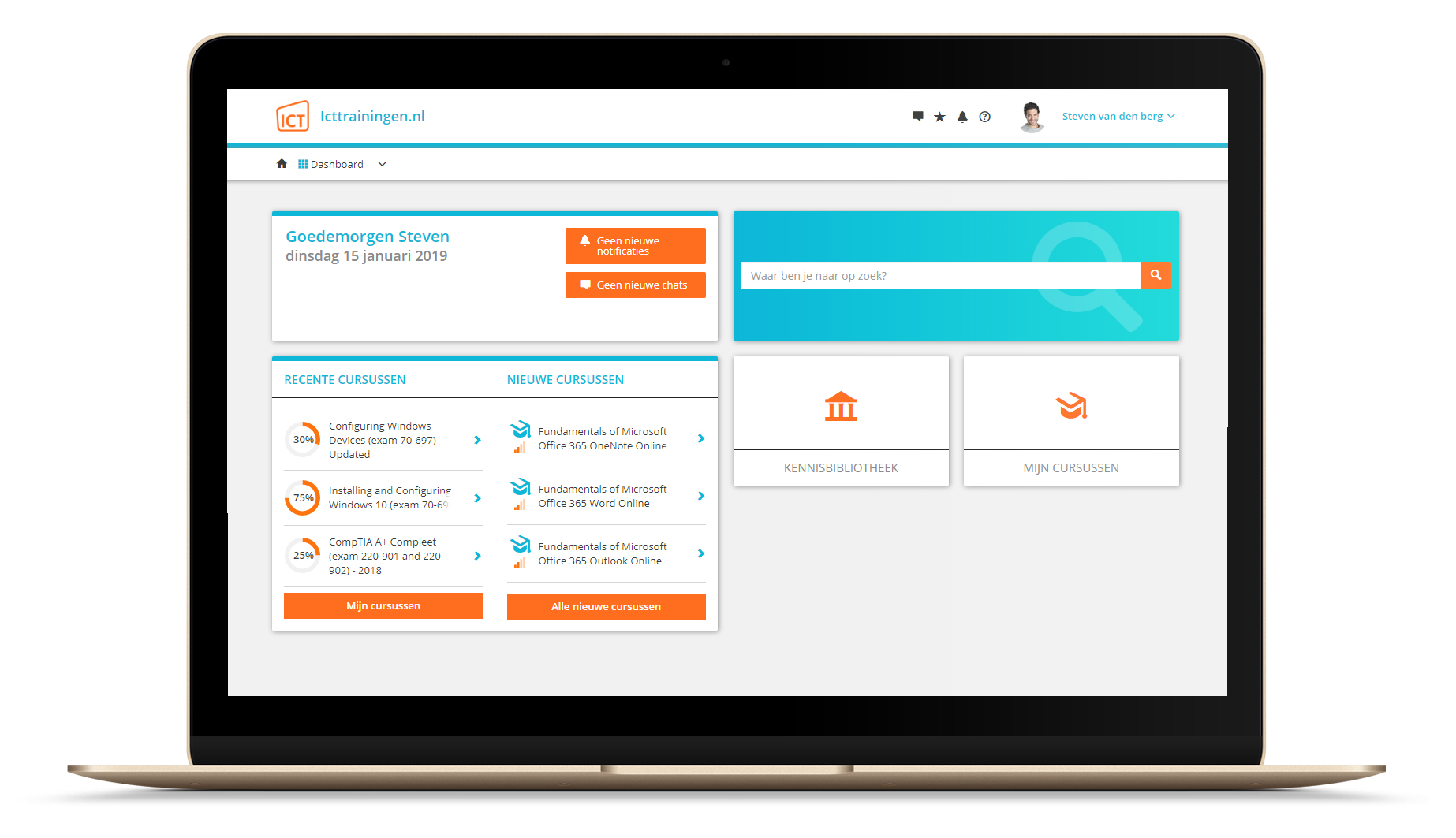
FAQ
Niet gevonden wat je zocht? Bekijk alle vragen of neem contact op.